Is there a quick and easy way to get the first character from a string in Python? Yes, there is using the slice operator.
To get the first character in a string simply use the code
my_string[0]
where my_string is a variable containing the string and the slice operator
[0]
captures the first index of that string, being the first character.
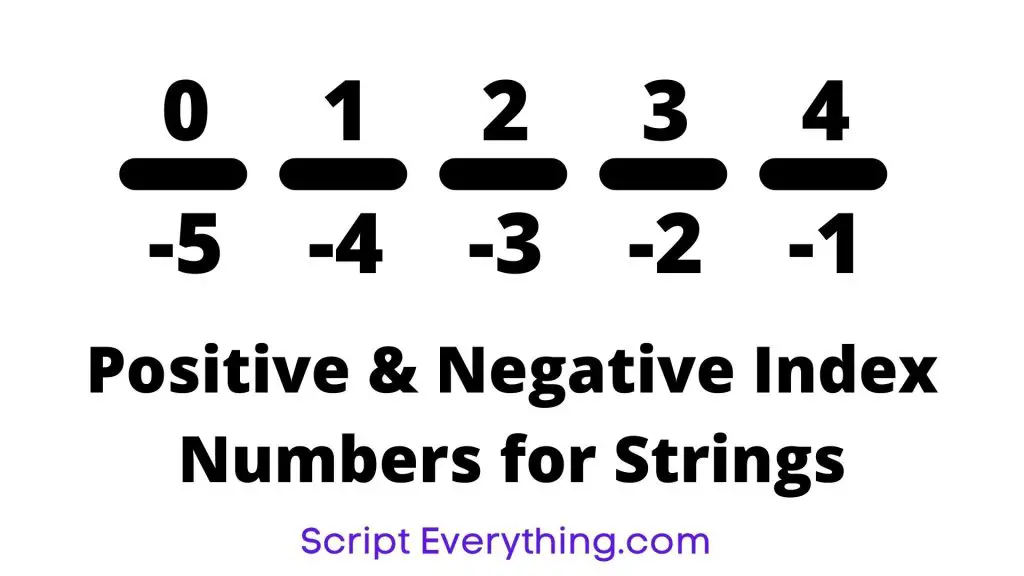
Here is an example demonstrating the use of this technique:
>>> my_string = "Uncopyrightable" >>> my_string[0] 'U'
As the capital U is the first character in the string this is what gets returned using the index reference of the string.
Why Can Characters In Strings Be Referenced By Index?
The reason why a string can be referenced by index in Python is that a string is an
iterable object
meaning the string class contains either an
__iter__()
method and/or a
__getitem__()
method. The latter method
__getitem__()
is the equivalent of using the square brackets with a number inside, like
[0]
.
Here’s how you can check if a variable contains the required
__getitem__()
method:
>>> my_string = "Are we an item?"
>>> type(my_string)
<class 'str'>
>>> '__getitem__' in dir(my_string)
True
>>> my_string.__getitem__(0)
'A'
As you can see from the above example the variable
my_string
is assigned the value
"Are we an item?"
which means the variable has instantiated a new instance of the
str
(string) class.
Is the
str
class an iterable? One way to check is by checking if the
__getitem__()
method is available on the class and one way of being able to obtain all the methods on a class is to use the built-in
dir()
function.
As the result from this function returns
True
you can use the method
__getitem__()
or its equivalent
[n]
on the variable to obtain the index position of an element on that variable.
Would this work for another data type such as an integer?
>>> i = 123456
>>> type(i)
<class 'int'>
>>> '__getitem__' in dir(i)
False
>>> i.__getitem__(0)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'int' object has no attribute '__getitem__'
>>> i[0]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not subscriptable
As you can see from the above example you cannot get the first character from a variable if the variable is from the class
int
. It does not contain the method
__getitem__()
on the class and therefore you are unable to fetch the first character from an integer number using the same technique you would otherwise using strings.
How To Use
__getitem__()
With Strings
The
__getitem__()
method on the string class is another way of being able to get the first character from a string.
The
__getitem__()
method takes one parameter being the reference to an index (in the case of variables such as lists, strings or tuples) or a key (in the case of dict) from the variable.
To use the
__getitem__()
method on a string data type variable simply insert the index reference number you want to get the character from that string.
For example, to get the first character from the string, use the index position
0
which denotes
the first position
in a string:
>>> my_string = "Uncopyrightable"
>>> my_string.__getitem__(0)
'U'
Notice in the example above how the same outcome is achieved using this method on the string class. To get the first character the index reference 0 is inserted into the
__getitem__
method.
Summary
There are a couple of ways to get the first character from a string both of which are equivalent. The shortest code possible is to use
my_string[0]
where
my_string
is a variable that has been assigned to the string to capture the first character from and the bracketed expression
[0]
uses the index reference 0 denoting the first item in the string.
The more verbose way of doing the exact same task is to use the
__getitem__(0)
method.
Now that you know how to capture the first character from a string you might also want to find out how to capture the last character from a string too.