How do you get the last character from a string using Python? Thankfully Python has an easy way to fetch the last character from a string using the slice operator .
To get the last character of the string in Python use the syntax
my_string[-1]
. The square brackets are used to indicate a specific character by index number, and as string data types can be iterated, you can use the
slice operator
[:]
, specifically
-1
inside to capture the last character.
Here’s the code:
my_string[-1]
And here’s a quick demonstration this in operation:
>>> my_string = "How long is a piece of string?"
>>> my_string[-1]
'?'
As can be seen from the above code, the final character in the example string used is the question mark.
This type of operation used on the original string is known as slicing as it uses the slice operator .
What Is The String Slice Operation?
The
slice operator
has the format
[start:stop:step]
where
start
is the first
index
item to start capture (
inclusive
),
stop
is the ending
indexed
item to stop capture, and
step
is the step or jump taken from the starting index to the ending index number.
Both
stop
and
step
are
optional
terms in the expression. If the
stop
parameter is empty the operation captures from the
start
to the
end of the string
. If the
step
parameter is empty the operation uses
1
as the step value.
Therefore, if
start
is the
only
parameter defined, for example,
[-1]
the slice operator starts its capture from index
-1
which is the last character of a string. As the
stop
parameter is empty, it will capture to the end of a string, returning just the last character.
Use Positive Indexes
You don’t have to use the slice notation on your strings with
-1
index reference. You can also use the slice operator with positive indexes, however, it is more code to obtain the last index number of your string.
As you need to find the length of your string, you will need the help of the
len()
function and then by subtracting
1
from this result, you’ll be converting it to an
index
number which the slice operator uses.
Here’s the code:
my_string[len(my_string)-1]
Here’s why you need to subtract 1 from the total length of the string, notice the index number of each character in the Python string:
Conversely, if we do the same operation but use a negative number in the slice operator, here’s what you will get:
Notice also in the last video the use of the function
range()
. This
range(start, stop, step)
function is the shorthand equivalent to the slice operator
[start:stop:step]
As you’ve seen from both demonstrations of positive and negative indexes you can easily extract the last character in a string using
[-1]
with a negative index, or, if you wanted to extract the last character using a
positive
index, it would be the length of the string minus 1.
A visual representation of the index number of a string can be seen from the below picture:
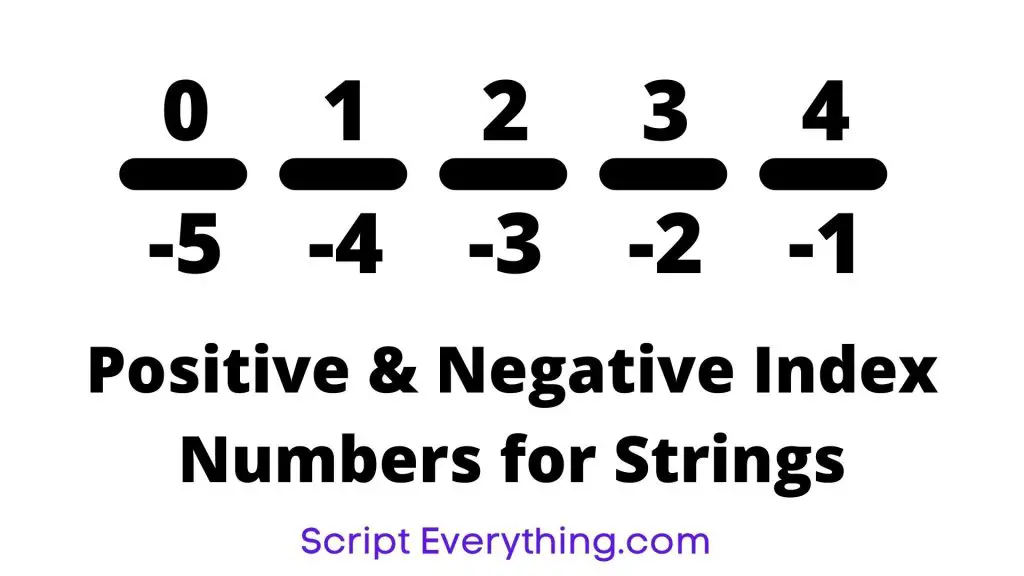
What Happens When String Is Empty?
If you have an empty string and try to perform a slice operation on it you will get an
IndexError
error, as demonstrated:
To alleviate this error, you can apply several different approaches:
-
Use a boolean expression as an empty string is
False
-
Use a
try-except
block
The first approach can be quite easy to implement, but it should be encouraged to check the data type of the variable, and that is of a
str
data type, otherwise you will get
TypeError
errors.
You can do this by using
type(my_string) == str
, here’s an example:
if my_string and type(my_string) == str:
my_string[-1]
else:
print("my_string is an empty string")
The other approach is to wrap your code in a
try-except
block, but these tend to be more computationally expensive than the other alternative:
try:
my_string[-1]
except IndexError as e:
print("my_string is empty")
Here’s how both approaches work:
How To Get Last
n
Characters From String
Knowing a little on how the
slice operator
works you can apply your knowledge on extracting just the last
n
characters of a string (rather than just the last).
To use the
slice operator
on a string to fetch the last
n
characters would mean starting from the last nth character and capturing all other individual characters to the end.
Staying with the use of negative indexes, you can increase that number to start the capture further back from the end.
One use case I had for this technique was capturing the postcodes of physical addresses. A data set of addresses was written in the following format:
StreetNumber StreetName StreetType, Suburb STATE PostCode
For example:
102 Smith Street, Sydney NSW 2000
With this slicing technique using negative indexes, I was able to extract the post code by using this code:
>>> address = "102 Smith Street, Sydney NSW 2000"
>>> post_code = address[-4:]
>>> print(post_code)
'2000'
Here’s a demonstration of this code in action:
Notice, though, one little detail with the slice operator that can be easily missed.
Notice I didn’t write this:
post_code = address[-4]
As this would have just returned the
index
position of the 4th last character from the string, being
2
. To ensure I capture everything to the end I need to make the
stop
parameter empty, which is why
address[-4:]
is needed.
By setting the second parameter blank, it implies the capture will go all the way to the end, and because no value was used for the third parameter (the step number), it captures everything from the fourth last variable through to the end.
Get Last Character From String In Python
To fetch the last character in a string use the slice operator by using
my_string[-1]
where
my_string
is the variable storing the string you want to fetch the last character from.
This is a quick handy technique to help you perform other types of string manipulation.
To fetch the last
n
characters of the string using the slice operator instead of using -1 as the value use a negative
n
number according to the number of characters you want to capture. For example,
my_string[-5:]
will capture the last 5 characters in a string whose variable is
my_string
.
Next, you might want to explore our other post on how to get the first n characters of a string using Python .