If you want to know how many words your WordPress articles have you can open up each post individually and by clicking on the top left-hand corner’s information icon it will display the number of words.
For example, when I completed this post I was curious as to the quantity of words I wrote and upon clicking the information icon within that post was informed of the following data:
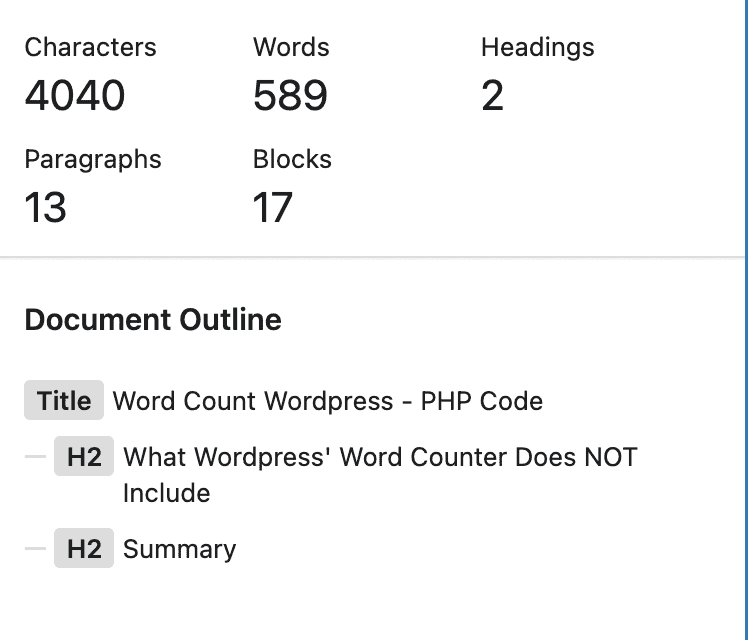
You can similarly obtain the same data from within the WordPress app if you have connected your WordPress instance with your Jetpack account. However, to obtain this information you would tap on the post and tap on the settings icon in the top right-hand corner.
At the top of the resulting menu displayed is the detail headed with Content Structure which lists for this post the following data:
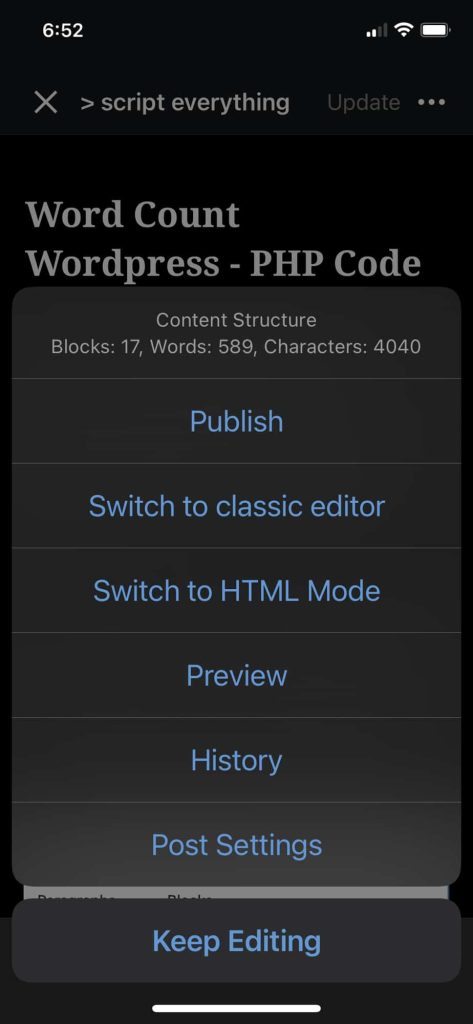
But can you get this information in the admin area of WordPress?
While this information is handy within the post is there a way we can display this data in our post listing within the admin area of WordPress?
Yes, but it will require some coding in the backend.
Simply navigate to your theme’s functions.php file and insert the following functions:
<?php
// Modify the columns in the Admin area of WordPress for both
// posts and pages.
add_filter('manage_posts_columns', 'my_site_display_wordcount');
add_filter('manage_pages_columns', 'my_site_display_wordcount');
/**
* Set the columns to display.
* @param $columns
* @return array
*/
function my_site_display_wordcount($columns) {
// create your own arrangement of columns, keeping `cb`, `title`, `date` and `categories`
// but changing their order and placement.
$columns = array(
'cb' => $columns['cb'],
'title' => $columns['title'],
'content_wordcount' => __('Content Count'),
'excerpt_wordcount' => __('Excerpt Count'),
'date' => $columns['date'],
'categories' => $columns['categories']
);
return $columns;
}
/**
* Counts the number of words in the text.
* @param $text_to_count
* @return number
*/
function my_site_count_words($text_to_count) {
return str_word_count(strip_tags($text_to_count));
}
// Perform the count for each row in the posts and pages admin tables
add_action('manage_posts_custom_column', 'my_site_word_count', 10, 2);
add_action('manage_pages_custom_column', 'my_site_word_count', 10, 2);
/**
* Set the word count.
* @param $column str
* @param $post_id number
* @return void
*/
function my_site_word_count($column, $post_id) {
if ($column == 'content_wordcount') {
$content = get_post_field( 'post_content', $post_id );
echo my_site_count_words( $content );
}
if ($column == 'excerpt_wordcount') {
$excerpt = get_post_field( 'post_excerpt', $post_id );
echo my_site_count_words( $excerpt );
}
}
And here’s how your posts table inside WordPress will look once this code is saved at the bottom of your functions.php file:

What WordPress’ Word Counter Does NOT Include
There are a couple of things the word count does not include in its calculations any of the following:
-
Numbers
– if you use numeric figures in your blog post these are not
words
.
- For example, the text: The year 2021. Is only counted as two words, not three.
- Code – this is kind of counted , depending upon the structure of your code and the spacing you place between your variables. For example, if you enter i = i + 1 this counts as 2 words, whereas its shorter cousin i += 1 only counts as 1 word. Any comments in your code do count towards the word count, so maybe this is a good thing to do if you’re trying to increase your word count – write lots of comments to help explain your code!
- Excerpts don’t count towards the posts’ content and therefore aren’t included in the count. This is why we decided to add them to our function above.
- Title of your blog post does not count towards the content of your blog post.
- Any tags inserted into the post do not count towards the word count total of your post.
- Any categories selected about the post do not count towards the word count of your post.
Summary
If you’re looking to report the amount of words in your blog post on the WordPress admin screen then you create some simple PHP code that can either be manually inserted into your theme’s functions file (not recommended), or you can create your own plug-in and import that code instead.