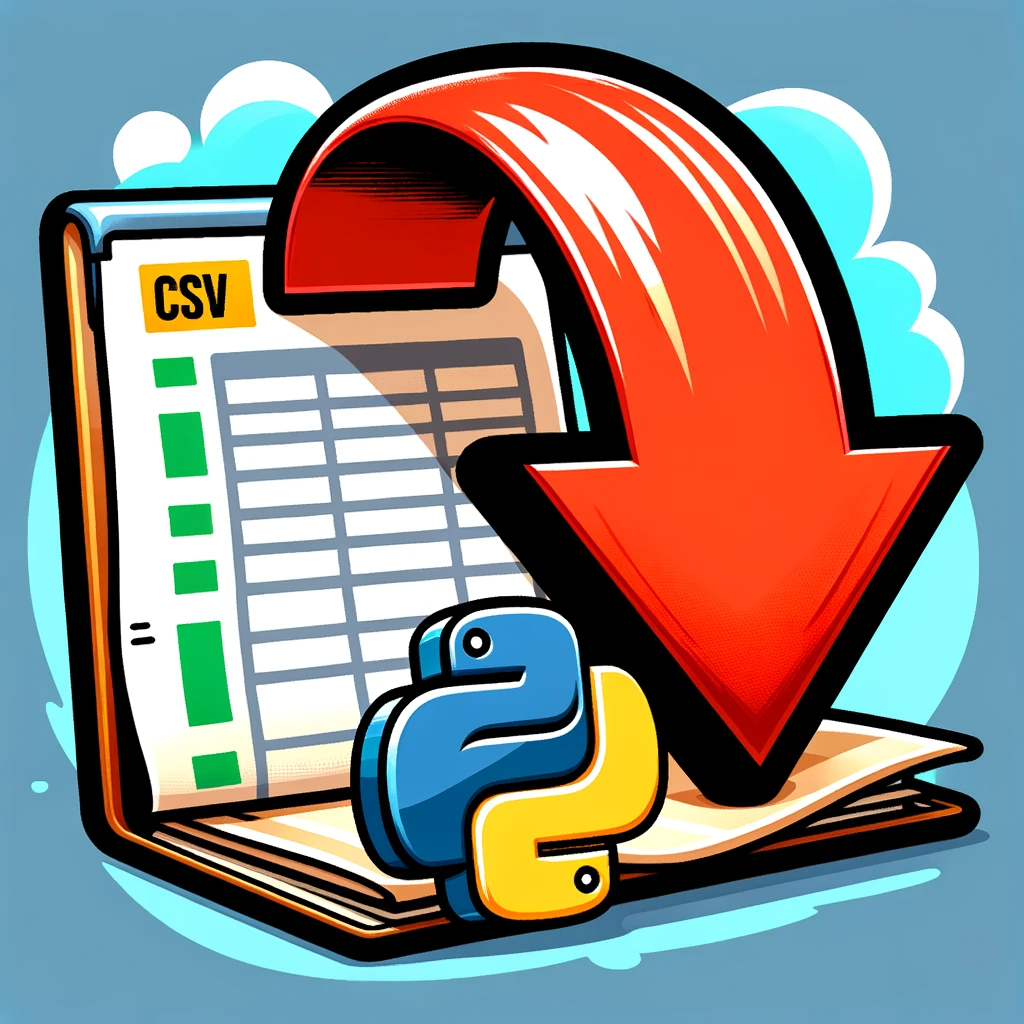
Read A CSV File To List Of Dictionaries In Python
The most common form of data I have played with is generally found in CSV format. This makes the data easy to understand but difficult to modify or change, especially when the CSV file contains lots of columns. In this article I will demonstrate how I frequently use this file format to import it into a useable data structure in Python, namely, a list of Python dictionaries. Let’s start with defining the basic terminology so we’re on the same page before jumping into some examples. ...