How do you create a radio button in a Suitelet form?
To create a series of radio button elements in a Suitelet form you need to add each element of the radio series as distinct fields using the
.addField
function. The
id
property needs to be the same for each radio field and the
source
property needs to be unique for each element.
Here’s an example demonstrating what this would look like where the user is required to select the option of “Direct Debit” or “Credit Card”:
As you can see from the code above, the
id
is the same for both fields as this is how radio buttons are grouped in a Suitelet. When selecting one of these radio buttons, it will deselect the other. Also, notice that the
source
property
must be set
and
must be unique
for each radio button.
The
source
property will be the value returned when the radio button is queried with a
scriptContext.currentRecord.getValue('custpage_payment_method')
call.
To keep the radio buttons contained, I also created a
FieldGroup
labelled
Payment Method
that is used to help keep the radio buttons contained within a group.
Here’s how this looks:
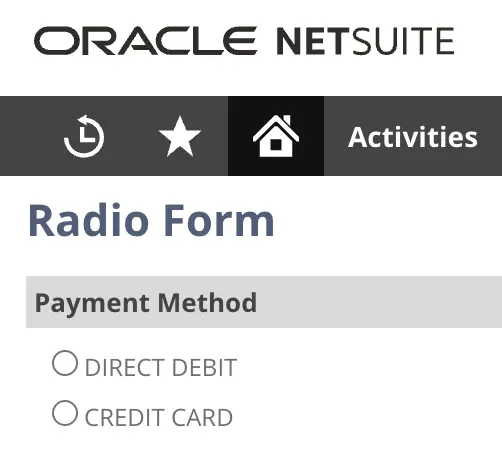
Here’s how this works when you select one of the fields:
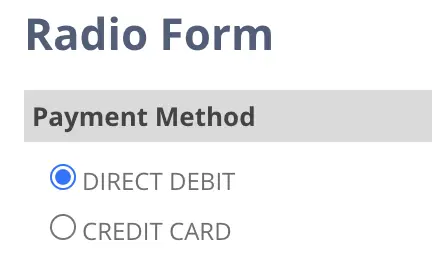
As the
source
property has
radio_dd
for its value when
scriptContext.currentRecord.getValue('custpage_payment_method')
is called (from the client script attached) this will return
radio_dd
.
And then when you select the other:
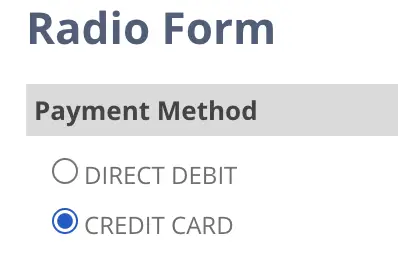
As you can see, when you select the other radio button field, it automatically deselects the other. Performing as a group of radio buttons should.
Similarly, as the
source
property has the value
radio_cc
for the credit card option selected a call to the radio button’s
getValue()
will return
radio_cc
.
Inserting Radio Into Sublist
If you are looking to insert a radio field into a sublist you only need to create the field once for each column.
Here’s a sample of what this would look like if your code needs the radio button in a sublist:
// ...
/** @type {Sublist} */
const mySublist = form.addSublist({
id: 'custpage_my_sublist',
label: 'My Sublist',
type: serverWidget.SublistType.LIST
});
/** @type {Field} */
const radioField = mySublist.addField({
id: 'custpage_sl_radio',
type: serverWidget.FieldType.RADIO,
label: 'Select',
source: 'radio_btn'
});
// ...
When you are populating your sublist with data there isn’t a need to individually insert for each line the radio field, this will automatically be added for you.
Then on the client-side script you can loop through the sublist to find which row has been selected.
Here’s an example on the
saveRecord()
function on the client-side script that helps to find which row was selected and to the perform further operation on obtaining the data from that selected row:
/**
* @param {Object} scriptContext
* @param {Record} scriptContext.currentRecord
* @returns {boolean} Return true if record is valid
*/
const saveRecord = (scriptContext) => {
/** @type {Record} */
const c = scriptContext.currentRecord;
/** @type {Number} */
const lines = c.getLineCount({sublistId: 'custpage_my_sublist'});
for (let i = 0; i < lines; i += 1) {
/** @type {String} */
const isRowSelected = c.getSublistValue({sublistId: 'custpage_my_sublist', fieldId: 'custpage_sl_radio', line: i});
if (isRowSelected === 'T') {
// ... capture data from the selected row ...
// once collected you can look to break out of the for loop
break;
}
}
}
You can use this type of code to check that at least one record has been selected and if not to throw a warning:
EXCEEDED_MAX_FIELD_LENGTH
Error For Radio Field
If you get the error
EXCEEDED_MAX_FIELD_LENGTH
for your radio button field, it means the unique
source
property names you have written for your radio button is longer than 15 characters.
To correct the error simply locate where in your Suitescript Suitelet code you have added a radio button field and count the number of characters used in the
source
property and reduce it to 15 characters or less.
This radio button would cause this error because the
source
property of
this_unique_id_is_too_long
is more than 15 characters long:
/** @type {Field} */
const ccRadioField = form.addField({
id: 'custpage_payment_method',
type: serverWidget.FieldType.RADIO,
source: 'this_unique_id_is_too_long',
label: 'Credit Card',
container: 'fieldgroup_method'
});
Simply modify that property to less than 15 characters.
Radio Button in Suitelet Form: Summary
To add a radio button to your Suitelet Suitescript code use the
addField
function from the
form
variable, make the
id
property the
same for each radio button in the group
, set the
type
property to
serverWidget.FieldType.RADIO
, and make each radio button’s
source
property unique (without exceeding 15 characters).