How do you change a paragraph into a list item in Google Docs using Google App Script?
To convert a paragraph into a list item using Google Docs identify first the paragraph you want to modify, then obtain the
childIndex
of where the paragraph is and finally insert the list item using
insertListItem()
function.
Here’s how this process works going through step-by-step:
Locate Paragraph To Change
In your Google App Script code create a variable to capture the active document using
DocumentApp.getActiveDocument()
or open the document if you have the documentId using
DocumentApp.openById(docId)
.
Here is an example using the active document:
// get the current working document
const doc = DocumentApp.getActiveDocument();
In the example below I am opening the
Document
using the
.openById()
method:
// Google Document ID
const docId = "Google Doc Id Hash String";
// open the document
const doc = DocumentApp.openById(docId);
Next, capture the body of the
Document
and obtain all the paragraphs:
// body of the document
const body = doc.getBody();
// get all paragraphs
const paras = body.getParagraphs();
Now that you have an array of
Paragraphs
it’s a matter of looping through each to identify which one will be replaced with the list items.
If you were looking for a
List
you could simply replace the last line of code above with something like this:
// get all lists
const lists = body.getListItems();
Notice that the capture of all items from the body is somewhat the same, just different functions used to obtain the data needed.
Once you have your array of
Paragraphs
or
ListItems
then it’s a simple matter of identifying which one will be swapped out.
In my Google document a paragraph is labelled
{ListGoesHere}
, therefore, I would only need to read the text of each
Paragraph
and whichever matches my identifier is the one that I need to capture.
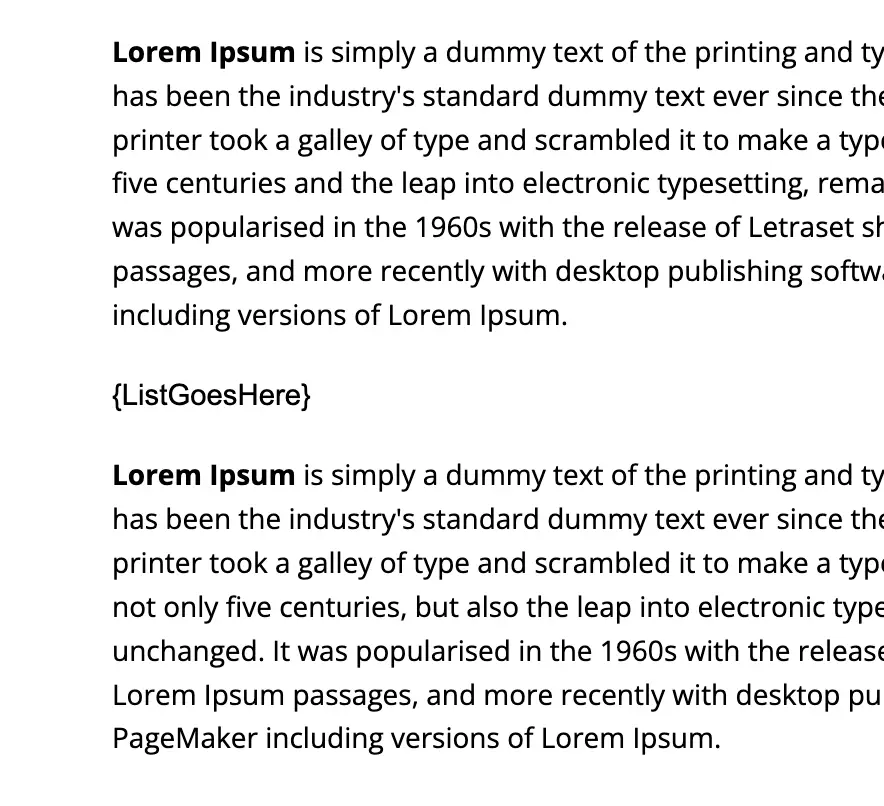
Here’s how this looks next:
// set what the identifying text is
const identifyingTag = "{ListGoesHere}"
// find paragraph matching the tag text
const matchedParas = paras.filter((el) => {
return el.getText().trim() === identifyingTag.trim();
});
To identify the tag in the paragraph text I have used the
getText()
method to capture all of the text in the paragraph and have further stripped any leading or trailing whitespace from the text. I’ve done the same to the
identifyingTag
variable as well (just in case!).
Using the
.filter()
array method helps to syphon through all the paragraphs in the document and to return only those matching the condition. It further means should there be
more than one paragraph
that matches the criteria the same matched paragraph will also change.
Now that you have identified the paragraph(s) to modify the last step is to make the modification.
Insert List Item Into Document
Currently, our working example has identified the paragraphs that match the criteria for where the list will be inserted. Now it’s a matter of inserting the list for each item we need.
The links to be inserted are from an external variable labelled
myListItems
, here is the code necessary to insert each of these text items into the
body
of the
Document
:
// list items to insert
const myListItems = ['Test 1', 'Test 2', 'Test 3'];
// choose glyph type for bullet point
const myGlyph = DocumentApp.GlyphType.HOLLOW_BULLET;
// loop through each identified paragraph and insert list
matchedParas.forEach((p) => {
const paraIdx = body.getChildIndex(p);
myListItems.forEach((li, idx) => {
body.insertListItem(paraIdx + idx + 1, li).setGlyphType(myGlyph);
});
// remove the original paragraph
body.removeChild(body.getChild(paraIdx));
});
The final section of the code above loops through each paragraph that matched the identifying criteria and then inserts list items and sets the type of list item to display. In my case I liked displaying the
HOLLOW_BULLET
and this was the glyph set for each bullet point.
As a result the text looked something like this:
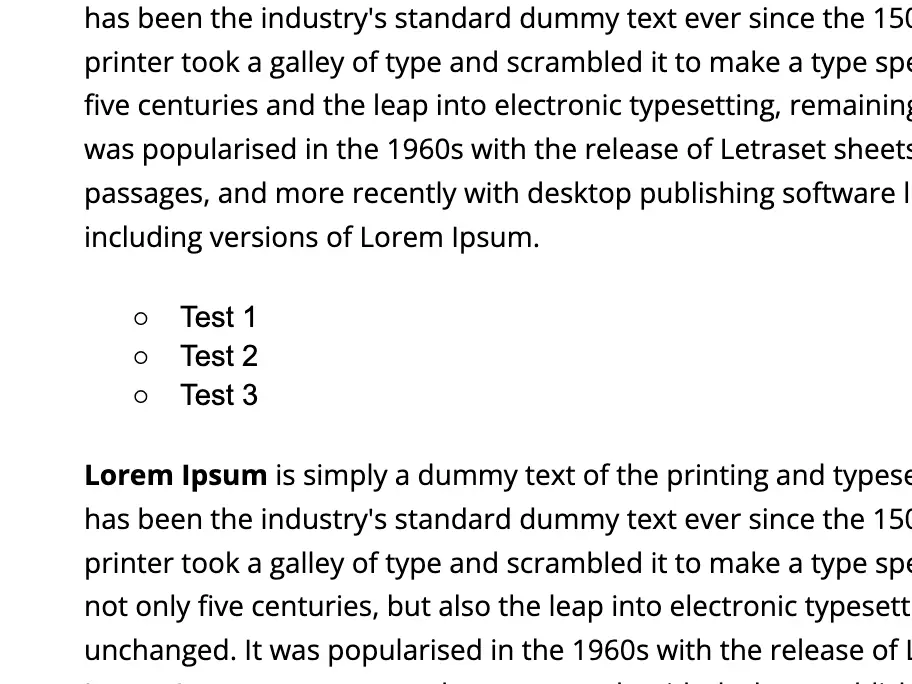
You can further chain other requirements to your list, for example, if each list were an object in the original
myListItems
variable that had not only the
text
to display but a
url
to link to you could further chain the method
.setLinkUrl(url)
so that the
.insertListItem()
line would look something like this:
// insert list item, set glyph and set url for whole list text
body.insertListItem(paraIdx + idx + 1, li['text']).setGlyphType(myGlyph).setLinkUrl(li['url']);
Summary
With Google App Script you can replace a paragraph with a list provided you can locate the contents of the paragraph you want to replace.
Once you’ve located the
childIndex
of the paragraph use the
insertListItem()
function and
setGlyphType()
accordingly for how you want the list to render in your Google Doc.